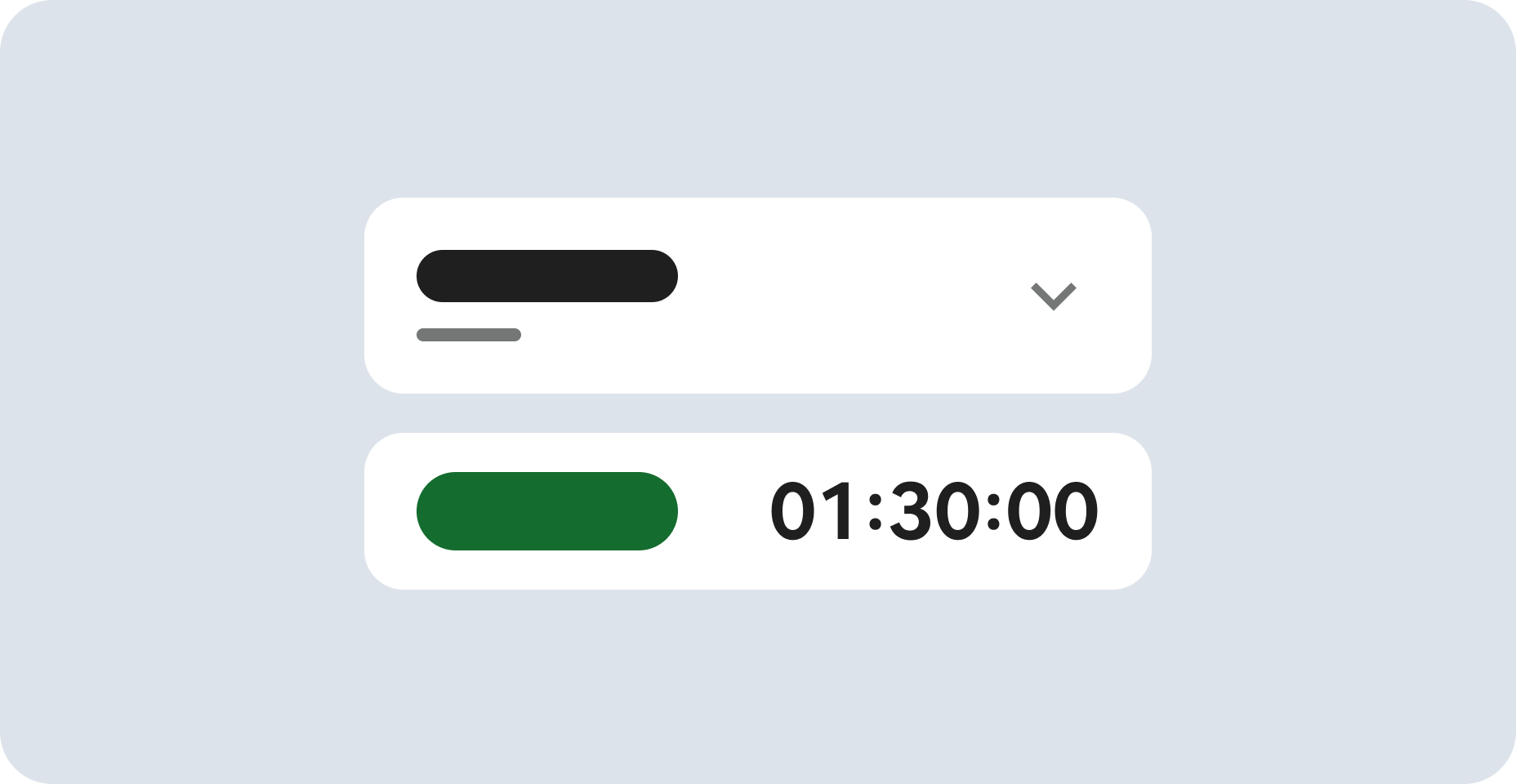
Before you begin
- Labs create a Google Cloud project and resources for a fixed time
- Labs have a time limit and no pause feature. If you end the lab, you'll have to restart from the beginning.
- On the top left of your screen, click Start lab to begin
An OpenAPI specification uses a standard format to describe a RESTful API. Written in either JSON or YAML format, an OpenAPI specification is machine readable, but is also easy for people to read and understand.
The specification describes elements of an API, including its base path, resource paths and verbs, operations, headers, query parameters, and responses. In addition, an OpenAPI specification is commonly used to generate API documentation.
In this lab, you will examine an OpenAPI specification for a retail backend service. You will then use this OpenAPI specification to create an API proxy that will be used to add features and security to the backend API.
In this lab, you learn how to perform the following tasks:
For each lab, you get a new Google Cloud project and set of resources for a fixed time at no cost.
Sign in to Qwiklabs using an incognito window.
Note the lab's access time (for example, 1:15:00
), and make sure you can finish within that time.
There is no pause feature. You can restart if needed, but you have to start at the beginning.
When ready, click Start lab.
Note your lab credentials (Username and Password). You will use them to sign in to the Google Cloud Console.
Click Open Google Console.
Click Use another account and copy/paste credentials for this lab into the prompts.
If you use other credentials, you'll receive errors or incur charges.
Accept the terms and skip the recovery resource page.
Google Cloud Shell is a virtual machine that is loaded with development tools. It offers a persistent 5GB home directory and runs on the Google Cloud.
Google Cloud Shell provides command-line access to your Google Cloud resources.
In Cloud console, on the top right toolbar, click the Open Cloud Shell button.
Click Continue.
It takes a few moments to provision and connect to the environment. When you are connected, you are already authenticated, and the project is set to your PROJECT_ID. For example:
gcloud is the command-line tool for Google Cloud. It comes pre-installed on Cloud Shell and supports tab-completion.
Output:
Example output:
Output:
Example output:
In this task, you will explore the OpenAPI specification that has been created for a backend service that you will use in your API proxies.
In Cloud Shell, download the OpenAPI specification for the backend service using this curl command:
This curl command downloads a file named retail-backend.yaml and stores it in a file with the same name in the home directory. Later in the lab, you will use this same specification when creating an API proxy.
In Cloud Shell, click Open Editor.
In the editor, select the retail-backend.yaml file.
Examine the OpenAPI specification.
This is the OpenAPI specification for the backend service that will be used in many of the course labs. Let's explore the sections of the OpenAPI spec.
The openapi field specifies the version of the OpenAPI specification. This is an OpenAPI version 3 spec, as indicated by the version number at the top of the file:
The info object provides metadata about the API itself. The version shown is the version of the Retail Backend specification:
The servers array contains a list of server objects that specify connectivity information for target servers. This specification contains a single backend service, which your API proxy will call:
The tags array adds metadata to tags used in the operations, which are shown below. Tags may be shared by multiple operations, and tags can be used to provide verbose descriptions or links to external documentation.
The paths object holds the relative paths to individual endpoints and their operations. One such path, /categories/{categoryId}, is used to specify a single category. Shown here is a get operation specified to get a category by ID. The get object shows parameters and responses. For operations that contain a request body, like PATCH /products/{productId}, the request body will also be specified.
The components object contains reusable objects for different portions of the OpenAPI spec. The securitySchemes component object contains definitions of different types of security schemes used by the operations. This spec defines a single Basic Authentication scheme, which is referenced in the PATCH /products/{productId} operation. The schemas component object contains input and output data types. Shown here is the Category object, which is returned when the GET /categories/{categoryId} operation returns successfully:
Feel free to explore the specification or the OpenAPI specification documentation.
Click Open Terminal.
Select Cloud Shell's More menu (), and then click Download.
Enter retail-backend.yaml
, and then click Download.
This will download the file to your local machine.
In this task, you will create an API proxy using the OpenAPI specification for the backend service.
In the Google Cloud console, on the Navigation menu (), look for Apigee in the Pinned Products section.
If Apigee is not in the Pinned Products section, to open the Apigee console page, type /
to start a search, then type Apigee
, and then, in the search results, click Apigee API Management.
The Apigee console page will open.
If Apigee is not pinned (), in the Navigation menu (), click Pin ().
The Apigee console page will now be pinned to the Navigation menu.
If the Apigee console page is not open, click Apigee.
On the left navigation menu, select Proxy development > API proxies.
To start the proxy wizard, click +Create.
For Proxy template, select OpenAPI spec template > Reverse proxy (Most common).
In OpenAPI specs, click Browse, select the retail-backend.yaml
file you downloaded, and then click Open.
Click Next.
Specify the following for the Proxy details:
Property | Value |
---|---|
Proxy name | retail-v1 |
Base path | /retail/v1 |
Description | My retail API |
The target was taken from the servers array in the OpenAPI spec. Leave the target unchanged.
Click Next.
Operations that were found in the OpenAPI specification are listed.
In Flows, on the header row, click Select all rows.
All flows should now be selected.
Click Next.
For Deployment environments, select the eval environment, and then click OK.
Click Create.
Your proxy will be generated and marked for deployment.
The full provisioning of an Apigee organization typically takes 30 minutes or longer. Most of the time is spent provisioning the runtime cluster, runtime database, and services that are used to run your API proxies. When creating a long-lived Apigee organization, this delay to full provisioning is not an issue. You do not, however, want to wait half an hour before starting each lab.
You will sometimes find that the organization is already fully provisioned when you enter the lab. Sometimes the Apigee organization will only begin provisioning when you start the lab.
The management plane operations of the organization are available after a few minutes of the provisioning process. Instead of waiting for the runtime to be fully provisioned, these labs allow you to perform operations like proxy editing before the runtime is available. When you deploy a proxy to an environment before the runtime is available, it will not be able to take traffic until runtime provisioning is complete.
When you hold the pointer over the Status icon for a deployed proxy, as shown below, you might see that there are no instances reporting status. This is normal until the Apigee organization's runtime has been fully provisioned.
A proxy that is deployed and ready to take traffic will show a green status on the Overview tab.
When a proxy is marked as deployed but the runtime is not yet available and the environment is not yet attached, you may see a red warning sign. Hold the pointer over the Status icon to see the current status.
If the proxy is deployed and shows as green, your proxy is ready for API traffic. If your proxy is not deployed because there are no runtime pods, you can check the provisioning status.
In Cloud Shell, to confirm that the runtime instance has been installed and the eval environment has been attached, run the following commands:
When the script returns ORG IS READY TO USE
, you can proceed to the next steps.
Select the Develop tab.
This tab is used to edit the proxy that has been generated. Conditional flows have been created for each of the operations in the OpenAPI specification. These conditional flows appear in the proxy endpoint in the Navigator on the left. When you click on a conditional flow, its request and response sections are selected in the visual editor pane. The default.xml
code shown below is the code representation of the proxy endpoint flows.
You will update many of these conditional flows in later labs.
Select the Debug tab.
The debug tool is used to trace API requests that are handled by the proxy.
Click Start Debug Session.
In the Start debug session pane, on the Environment dropdown, select eval.
The deployed revision number will also show in the dropdown.
Click Start.
The debug session will run for 10 minutes.
The eval environment in the Apigee organization can be called using the hostname eval.example.com. The DNS entry for this hostname has been created within your project, and it resolves to the IP address of the Apigee runtime instance. This DNS entry has been created in a private zone, which means it is only visible on the internal network.
Cloud Shell does not reside on the internal network, so Cloud Shell commands cannot resolve this DNS entry. A virtual machine (VM) within your project can access the private zone DNS. A virtual machine named apigeex-test-vm was automatically created for this purpose. You can make API proxy calls from this machine.
The curl command will be used to send API requests to an API proxy. The -k
option for curl tells it to skip verification of the TLS certificate. For this lab, the Apigee runtime uses a self-signed certificate. For a production environment, you should use certificates that have been created by a trusted certificate authority (CA).
In Cloud Shell, open a new tab, and then open an SSH connection to your test VM:
The first gcloud command retrieves the zone of the test VM, and the second opens the SSH connection to the VM.
If asked to authorize, click Authorize.
For each question asked in the Cloud Shell, click Enter or Return to specify the default input.
Your logged in identity is the owner of the project, so SSH to this machine is allowed.
Your Cloud Shell session is now running inside the VM.
To make a call to proxy hosted in the eval environment, in the Cloud Shell SSH session, send a request to your API proxy by using this command:
A transaction for this request should appear in the Transactions pane on the left. When a transaction is selected, you'll see a trace of the request and response through Apigee. You should see a 200 status code if your backend URL was correctly set and you correctly updated the Send Requests URL.
Click the Back and Next buttons to navigate through the steps of the transaction.
The request was GET /retail/v1/categories
. This request was sent to the backend, which responded with a JSON array containing the categories.
In this lab, you learned about OpenAPI specifications, and explored some of the features in OpenAPI specs. You used an OpenAPI specification for a retail backend service to create an API proxy, and you traced calls through that proxy.
When you have completed your lab, click End Lab. Google Cloud Skills Boost removes the resources you’ve used and cleans the account for you.
You will be given an opportunity to rate the lab experience. Select the applicable number of stars, type a comment, and then click Submit.
The number of stars indicates the following:
You can close the dialog box if you don't want to provide feedback.
For feedback, suggestions, or corrections, please use the Support tab.
Copyright 2022 Google LLC All rights reserved. Google and the Google logo are trademarks of Google LLC. All other company and product names may be trademarks of the respective companies with which they are associated.
This content is not currently available
We will notify you via email when it becomes available
Great!
We will contact you via email if it becomes available
One lab at a time
Confirm to end all existing labs and start this one